WAM
What is WAM
If your function or command requires user interaction or needs to show specific information to the user, you can create and use a Web Application Module (WAM).
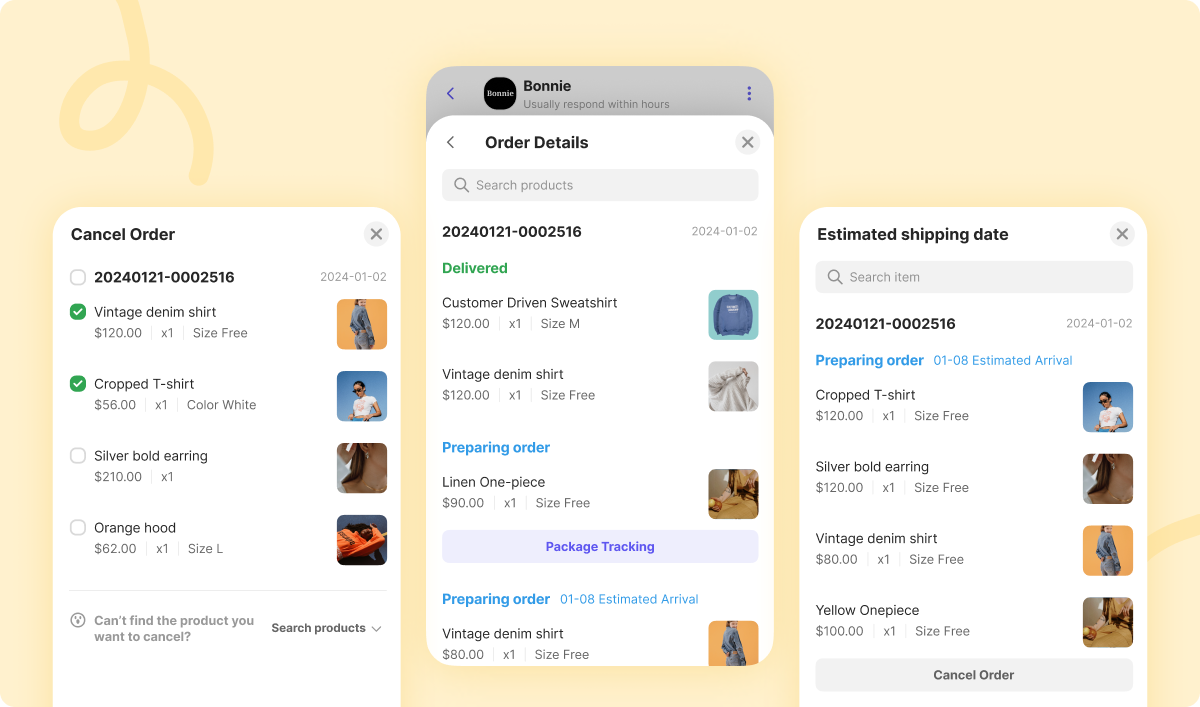
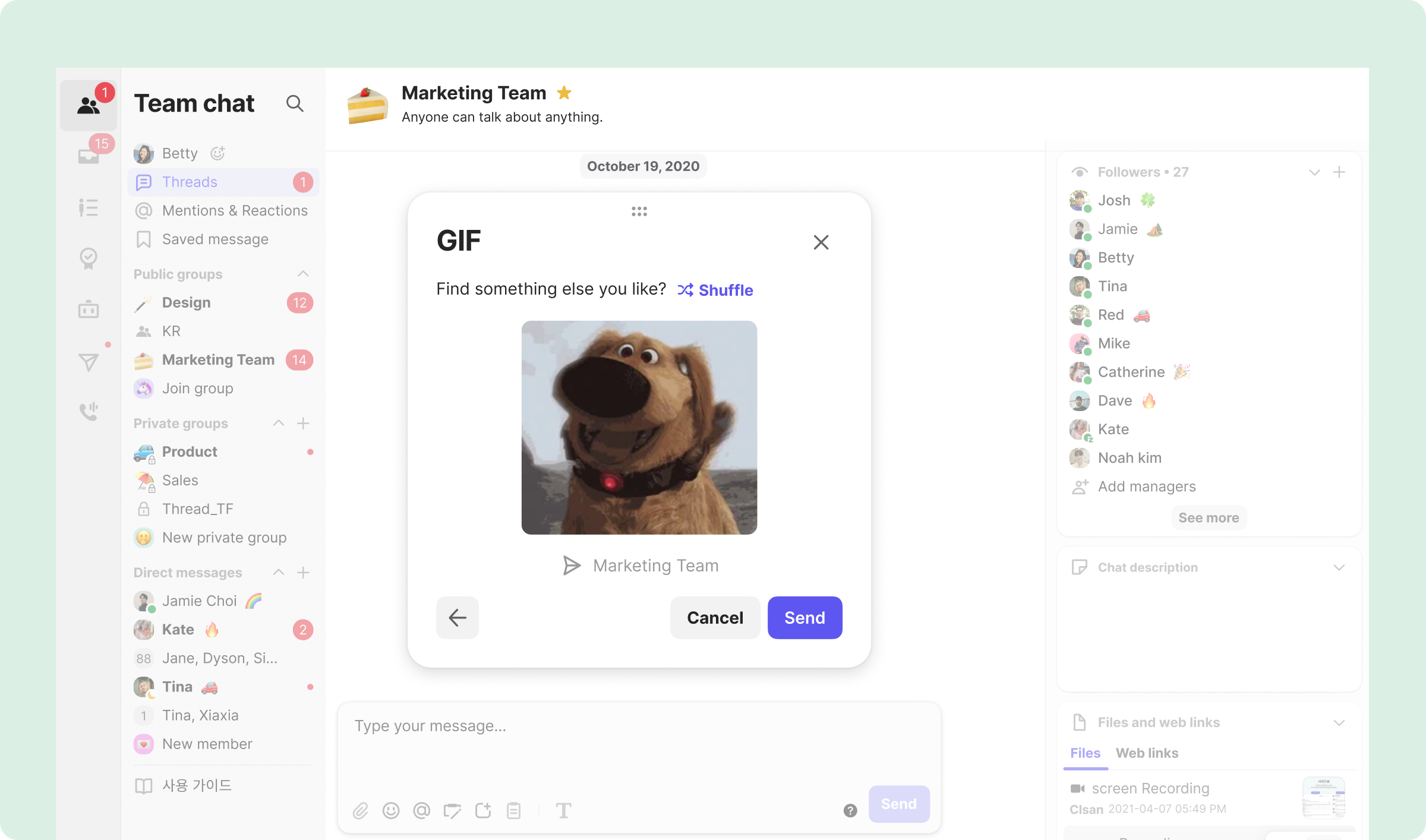
WAM should be implemented as a single-page application (SPA). The library, framework, bundler, etc. for implementing WAM is the developer's choice, and any choice is fine.
You can register WAMs by making them serve on a sub-endpoint of your app server domain and entering the endpoint URL in the 'WAM Endpoint' field in the app setup step.
For example, if the wamName
of your WAM is example-1-app
, the server will call the URL ${WAM_ENDPOINT}/example-1-app
.
WAM Controller
WAM looks like a single UI within the desk/front, but the execution itself takes place within a separate, isolated space, the WAM Controller.
The WAM Controller does the following to ensure that WAM runs smoothly
- Isolates WAM via an iframe, ensuring that WAM and the clients that run it (Desk, Front) each operate in a secure environment.
- Provides the necessary interfaces for WAM to operate.
When developing WAM, you can implement various functions using the interfaces provided by WAM Controller. In addition, you need to use the functions provided by WAM Controller to resize WAM, implement normal close behavior, etc.
The interfaces provided by WAM Controller are available through the ChannelIOWam
namespace.
interface Window {
ChannelIOWam: {
close: Close
setSize: SetSize
callFunction: CallFunction
callNativeFunction: CallNativeFunction
callCommand: CallCommand
getWamData: GetWamData
getVersion: GetVersion
version: string
}
}
The interface provided by WAM Controller is shown below.
close
Terminates the currently running WAM.
If you want to run a command at the same time as the shutdown, pass the command information as an argument.
Argument | Type | Description |
---|---|---|
appId | string | the ID of the app that provides the command you want to invoke |
name | string | the name of the command you want to invoke |
params | object | any parameters required by the command you want to invoke |
interface CloseArgs {
appId: string;
name: string;
params?: object;
}
type Close = (args?: CloseArgs = {}) => void;
If you simply want to close the currently running WAM, you do not need to pass any arguments.
window.ChannelIOWam.close()
If you want to close the currently running WAM and run a command, pass a value as an argument.
window.ChannelIOWam.close({
appId: 'EXAMPLE_APP_ID',
name: 'COMMAND_NAME',
params: {}
})
setSize
Resize the WAM in Desk.
Argument | Type | Description |
---|---|---|
width | number | width of the WAM to be changed |
height | number | height of the WAM to be changed |
interface SetSizeArgs {
width: number;
height: number;
}
type SetSize = (args: SetSizeArgs) => void;
window.ChannelIOWam.setSize({
width: 400,
height: 300,
})
callFunction
Call Function. For more information about Functions, see Guide - Function.
Argument | Type | Description |
---|---|---|
appId | string | the ID of the app that provides the function you want to call |
name | string | the name of the function you want to call |
params | object | any parameters required by the function you want to call |
interface CallFunctionArgs {
appId: string;
name: string;
params?: object;
}
type CallFunction = (args: CallFunctionArgs) => Promise<any>;
window.ChannelIOWam.callFunction({
appId: 'APP_ID',
name: 'FUNCTION_NAME',
params: {},
})
The following example demonstrates how to call the getUsers
function of an application with an appId
of EXAMPLE_APP_ID
and no parameters.
async function getUserList() {
const { result } = await window.ChannelIOWam.callFunction({
appId: 'EXAMPLE_APP_ID',
name: 'getUsers',
params: {},
})
return result.users
}
callNativeFunction
Call the native function. This allows you to read and modify Channel Talk data. For more information about Native function, see Guide - Function.
Argument | Type | Description |
---|---|---|
name | string | the name of the native function you want to call |
params | object | the parameters required by the native function you want to call |
interface CallNativeFunctionArgs {
name: string;
params?: object;
}
type CallNativeFunction = (args: CallNativeFunctionArgs) => Promise<any>;
window.ChannelIOWam.callNativeFunction({
name: 'FUNCTION_NAME',
params: {},
})
The following is an example of code to send a message to a specific group in Team Chat:
async function sendHelloToGroup() {
const channelId = window.ChannelIOWam.getWamData('channelId')
const chatId = window.ChannelIOWam.getWamData('chatId')
const managerId = window.ChannelIOWam.getWamData('managerId')
await window.ChannelIOWam.callNativeFunction('writeGroupMessageAsManager', {
channelId,
groupId: chatId,
rootMessageId,
broadcast,
dto: {
plainText: "Hello!",
managerId,
},
})
}
The following is an example of code that sends a message to a specific User Chat as an internal conversation:
async function sendPrivateMessageToUserChat() {
const channelId = window.ChannelIOWam.getWamData('channelId')
const chatId = window.ChannelIOWam.getWamData('chatId')
const managerId = window.ChannelIOWam.getWamData('managerId')
await window.ChannelIOWam.callNativeFunction('writeUserChatMessageAsManager', {
channelId,
userChatId: chatId,
dto: {
plainText: "This is private!",
options: ['MESSAGE_OPTION_PRIVATE'],
managerId,
},
})
}
callCommand
Call Command. For more information about Commands, see Guide - Command.
Argument | Type | Description |
---|---|---|
appId | string | the ID of the app that provides the command you want to invoke |
name | string | the name of the command you want to invoke |
params | object | any parameters required by the command you want to invoke |
interface CallCommandArgs {
appId: string;
name: string;
params?: object;
}
type CallCommand = (args: CallCommandArgs) => void;
window.ChannelIOWam.callCommand({
appId: 'APP_ID',
name: 'COMMAND_NAME',
params: {},
})
The following is an example of code that calls the order-cancellation
Command with params for an app with appId
EXAMPLE_APP_ID
:
function callOrderCancellationCommand(userId, orderId) {
window.ChannelIOWam.callCommand({
appId: 'EXAMPLE_APP_ID',
name: 'order-cancellation',
params: {
userId,
orderId,
},
})
}
Updated 8 months ago