Push Notification
You can integrate Channel Talk Android SDK with Firebase Cloud Messaging(FCM) to deliver push notifications when a manager replies to a message. Complete installing the SDK before following these steps.
To use system push notification in Android API level 33(Tiramisu) or above, you need POST_NOTIFICATIONS permission.
Channel Android SDK does not request permission. Your application should request it manually. Seeย Android official documentation regarding notification.
Firebase integration
Setting up a Firebase project
- Configure Android project with Firebase setup guide.
- Download the
google-service.json
file and place it in the root directory of the app scope module.
Integrating Firebase with SDK
Follow one of the two sections based on whether your project has Firebase imported.
If your project was not using Firebase
You can add the following dependency, which integrates with the FCM automatically. The dependency will automatically handle Firebase messages.
Declare our Maven repository to the build.gradle:
dependencyResolutionManagement {
repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS)
repositories {
mavenCentral()
maven { url 'https://maven.channel.io/maven2' }
}
}
Then add dependency to the project level build.gradle. See our change logs for the latest version.
dependencies {
// A core module
implementation 'io.channel:plugin-android:$[version]'
// Used to setup FCM easily
implementation 'io.channel:plugin-android-fcm:$[version]'
}
If your project was already using Firebase
You should forward some information to the SDK to process push notifications using ChannelIO.receivePushNotification()
.
class SampleFirebaseMessagingService : FirebaseMessagingService() {
override fun onNewToken(refreshedToken: String) {
ChannelIO.initPushToken(refreshedToken)
// ...
}
override fun onMessageReceived(remoteMessage: RemoteMessage) {
val pushMessage = remoteMessage.data
if (ChannelIO.isChannelPushNotification(pushMessage)) {
ChannelIO.receivePushNotification(application, pushMessage)
} else {
// ...
}
}
}
If FCM is already installed on your project,
onNewToken
might not be called. In this case, push notifications will not be received. Reinstalling the application will fix the issue.
In your appโs launcher activity, call openStoredPushNotification
to launch the associated chat with the notification.
class SampleActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
if (ChannelIO.hasStoredPushNotification(this)) {
ChannelIO.openStoredPushNotification(this);
}
}
}
Any notification-related information stored in the SDK is removed during boot. You should call
openStoredPushNotification
before your SDK completes the boot.
Integrate Firebase with Channel Desk
- Navigate to Project Overview > Project settings.
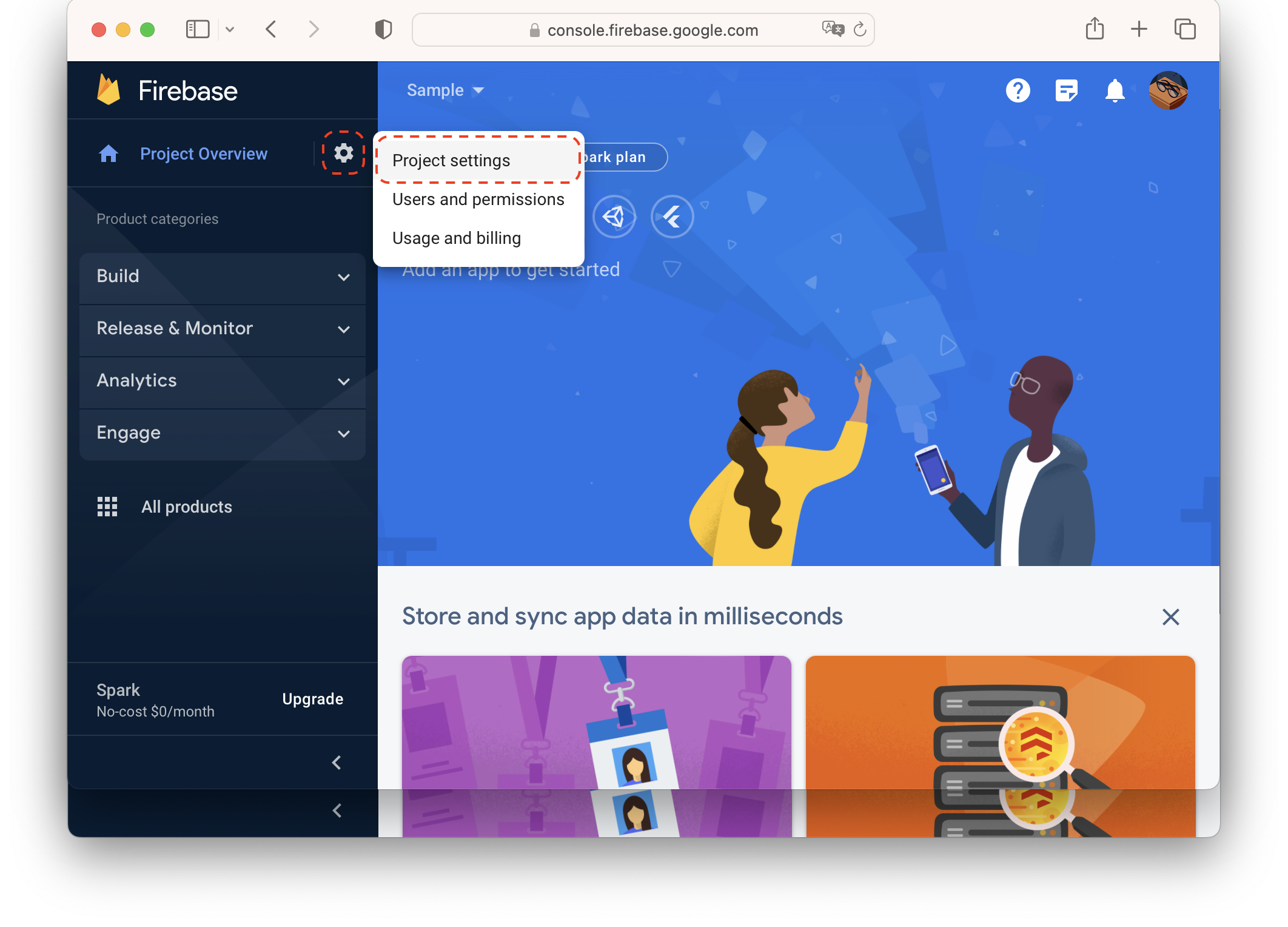
- Navigate to Cloud Messaging tab and click Manage Service Accounts.
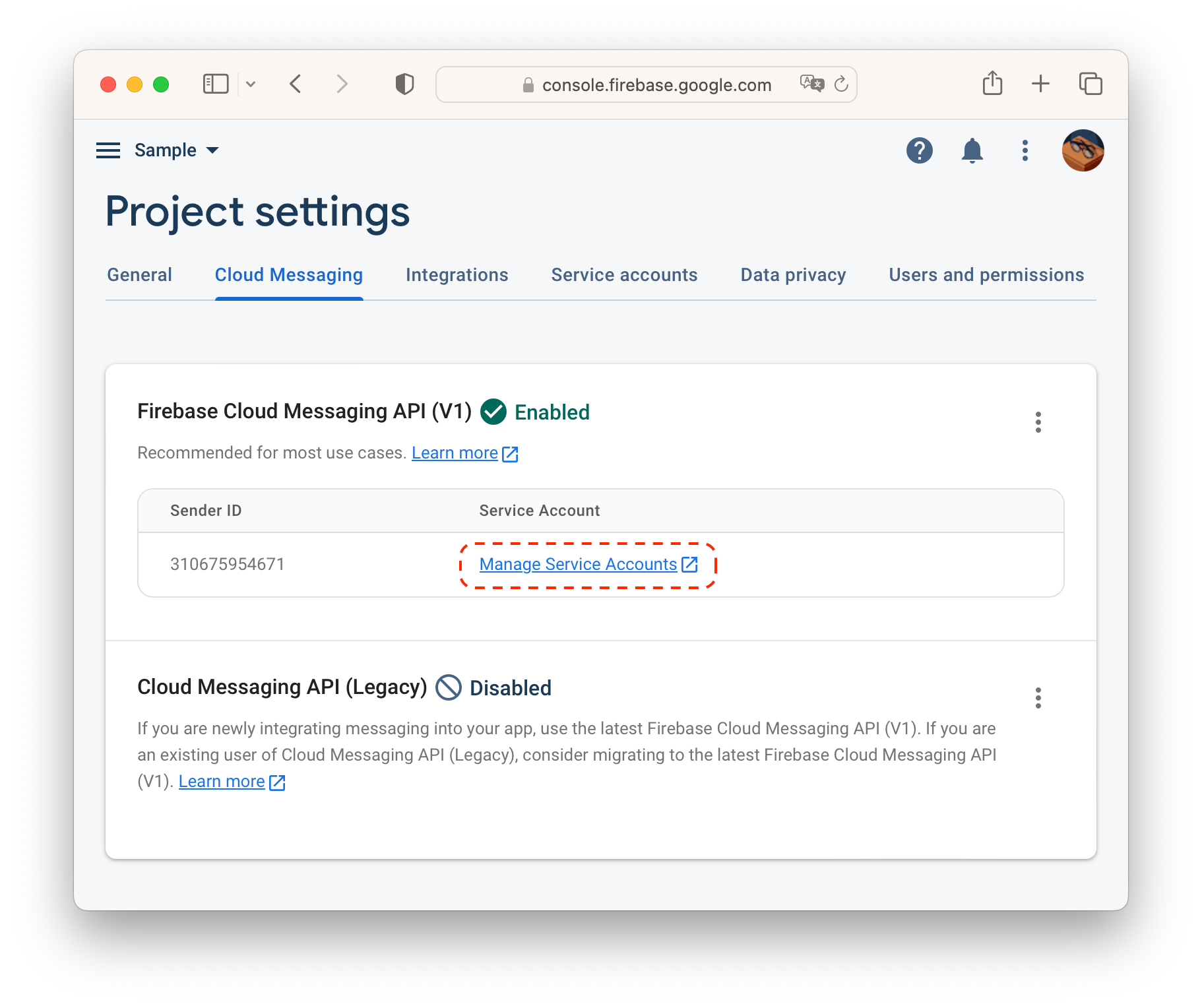
- Prior to creating a service account, create a role. Navigate to Roles.
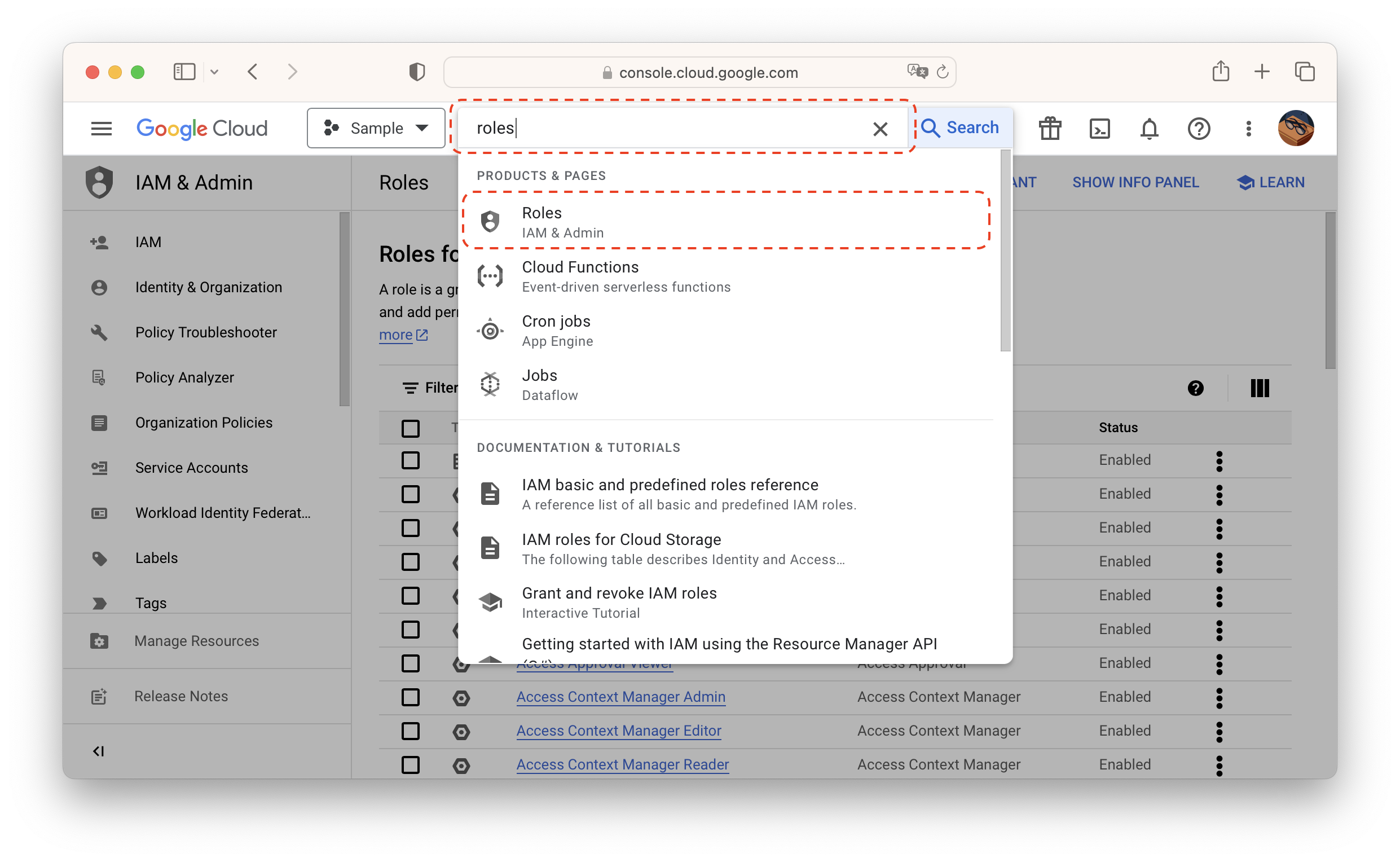
- Click CREATE ROLE.
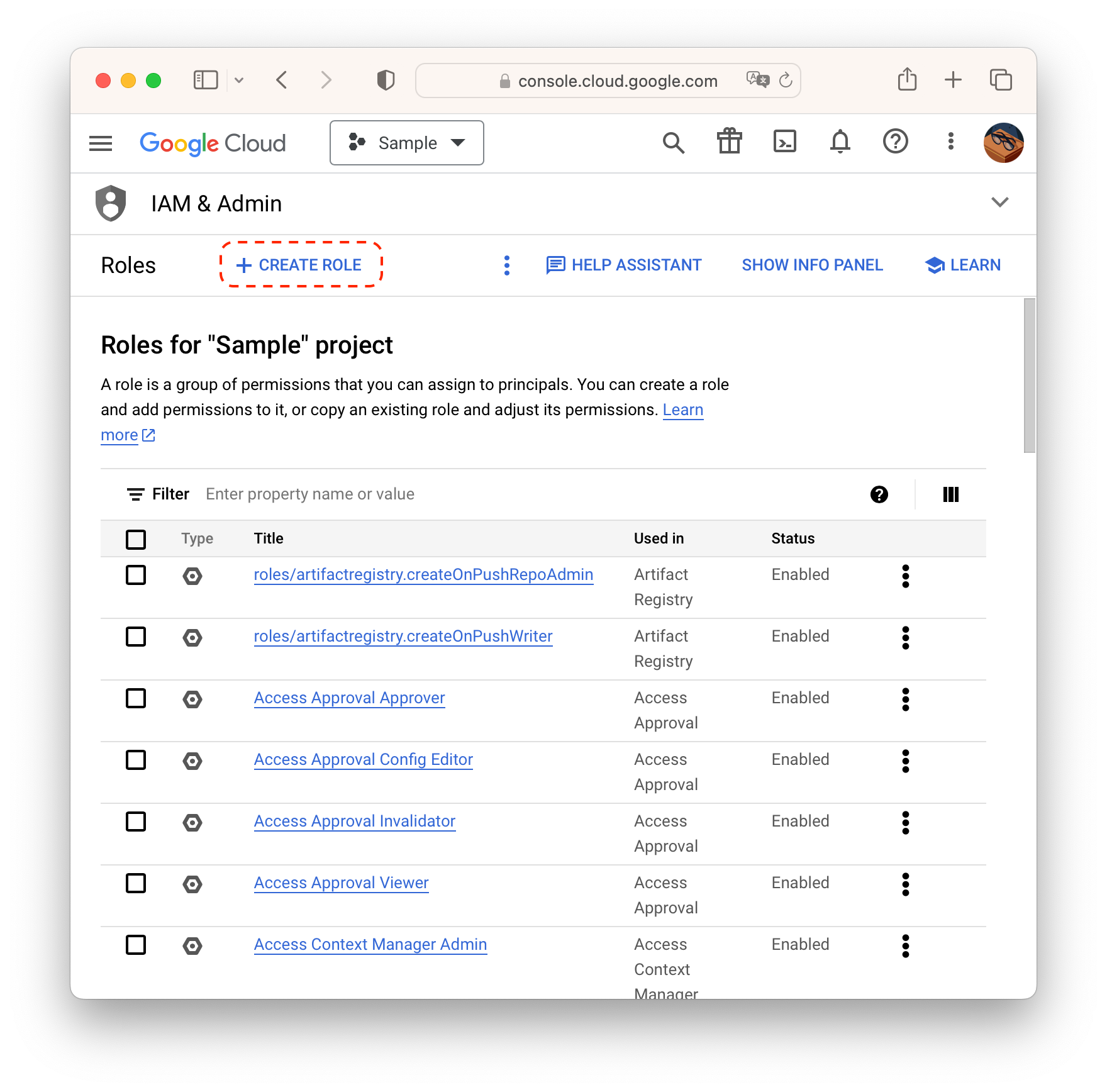
- Fill in details and click ADD PERMISSIONS.
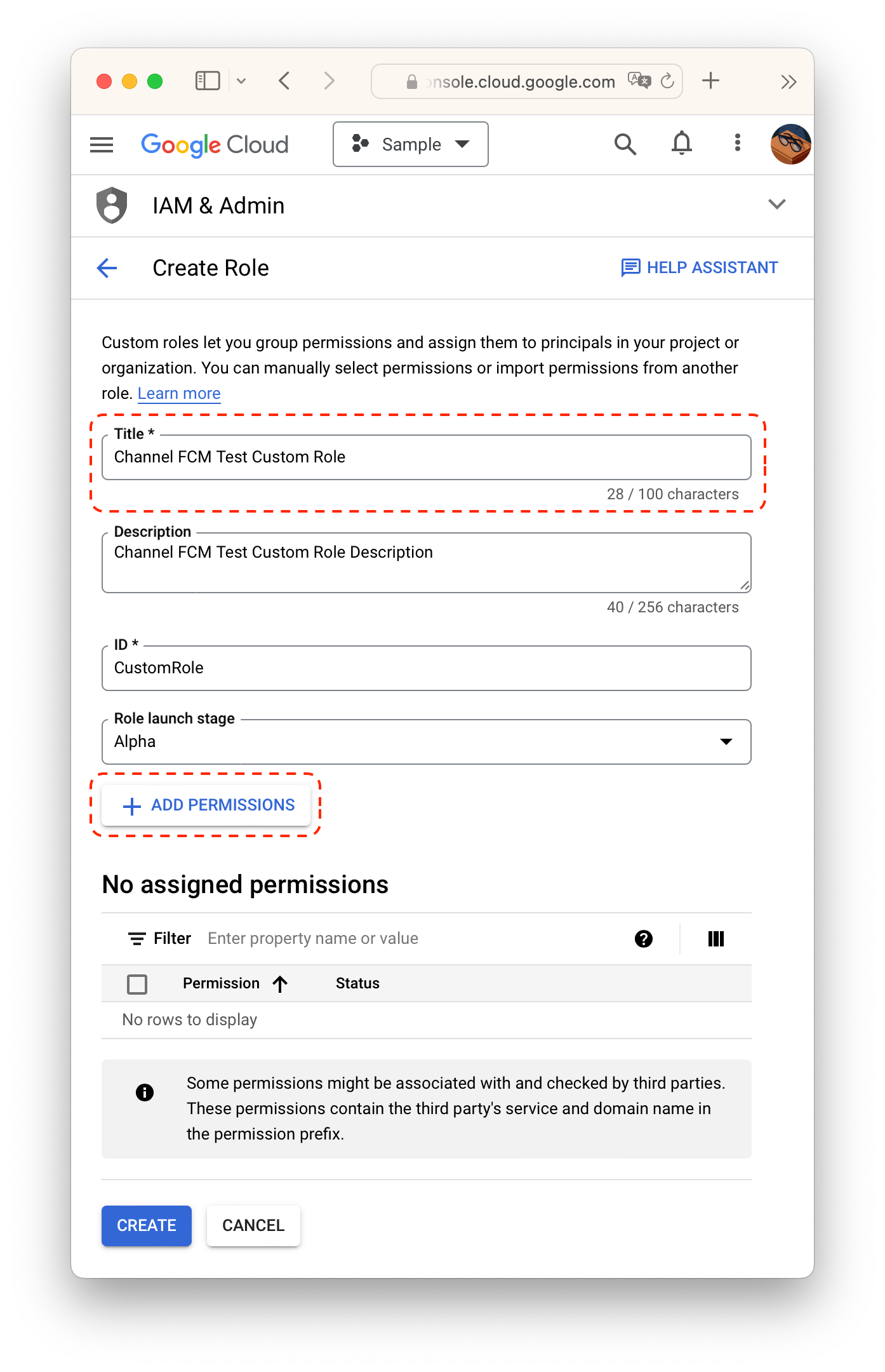
- Create a role with cloudmessaging.messages.create permission. You can find it by keyword using filter. Make sure you are typing keywords to "Enter property name or value" field NOT "Filter permissions by role."
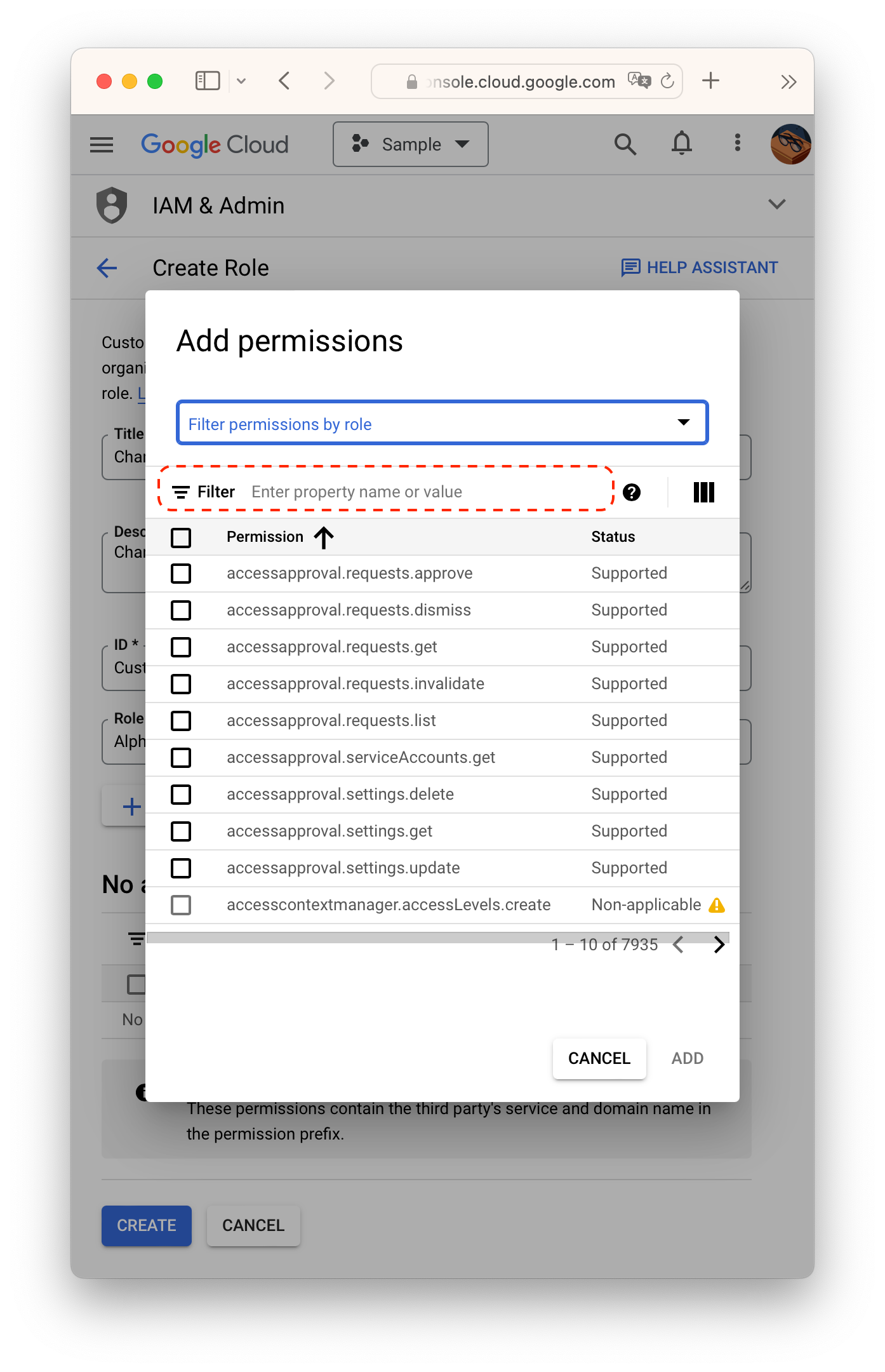
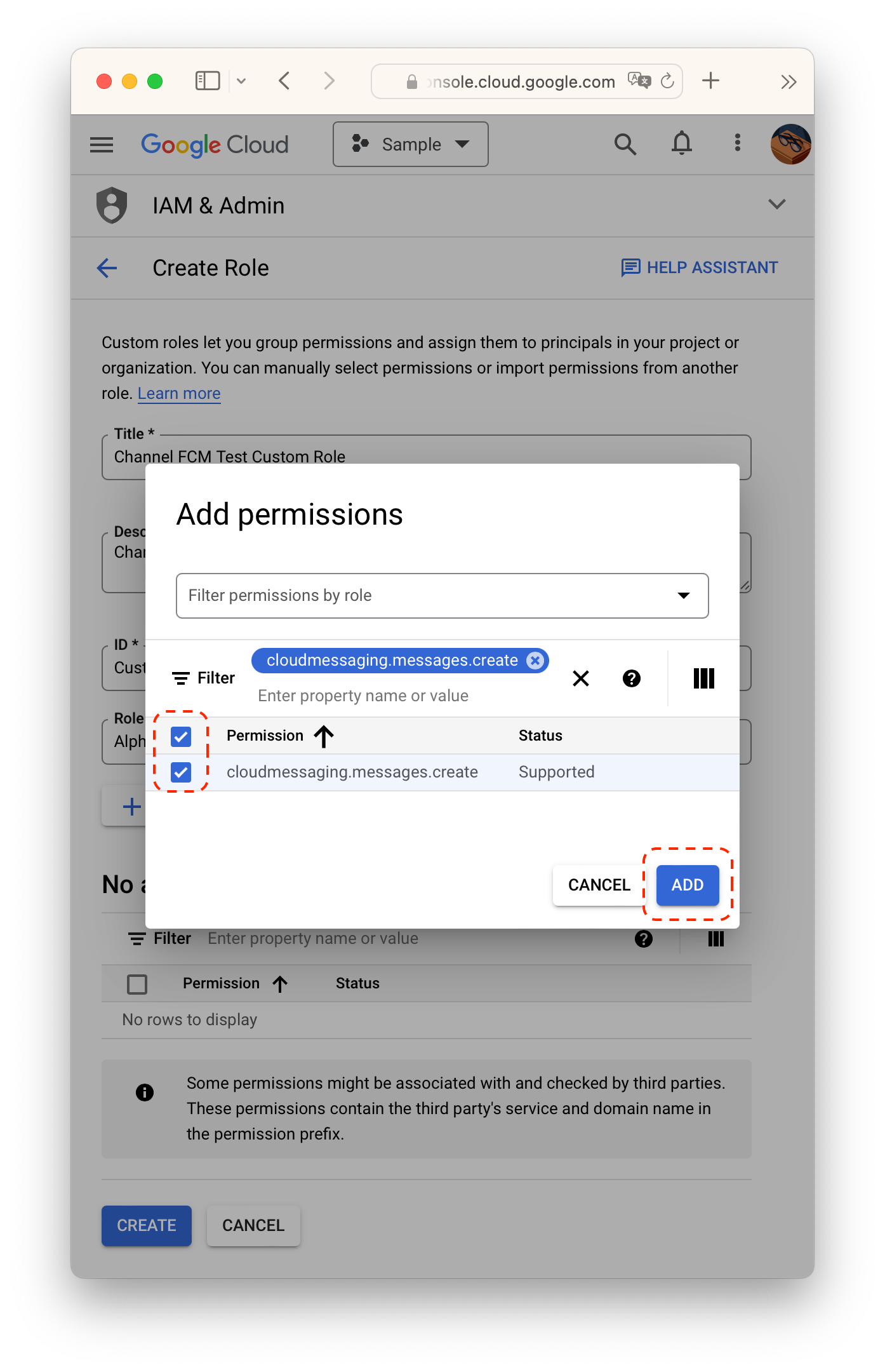
- Navigate back to service accounts page and click CREATE SERVICE ACCOUNT.
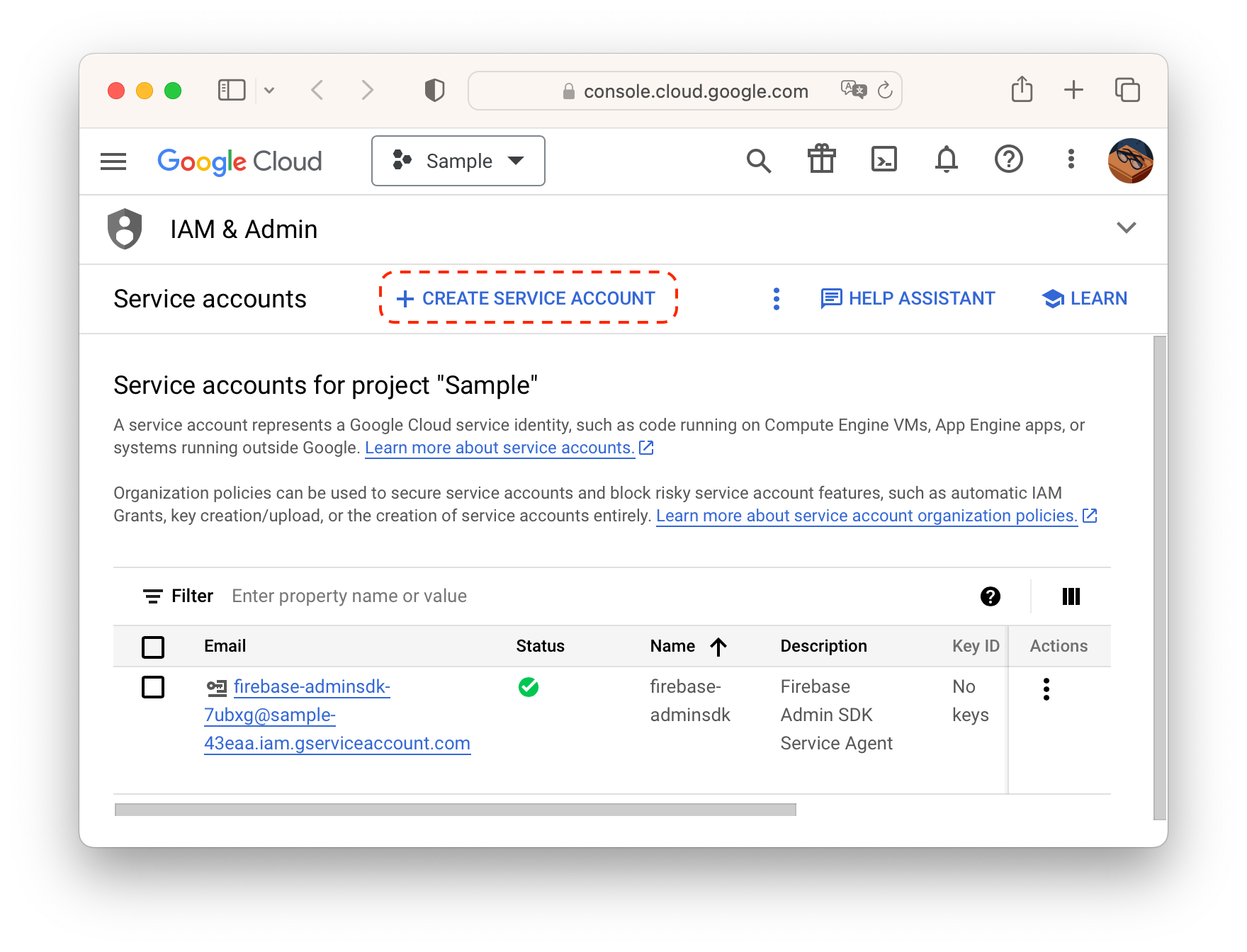
- Fill in details and click CREATE AND CONTINUE.
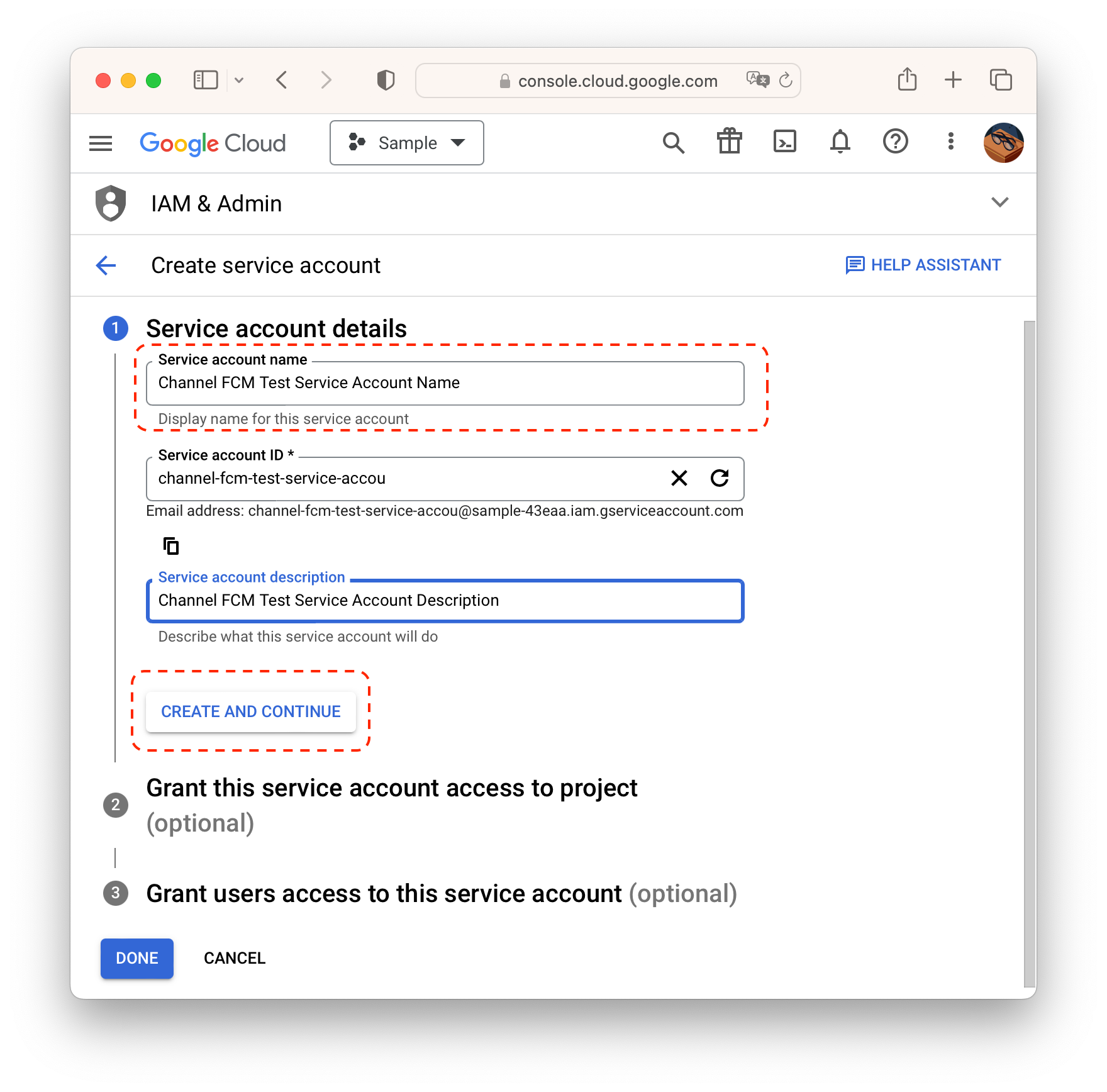
- Click Select a role.
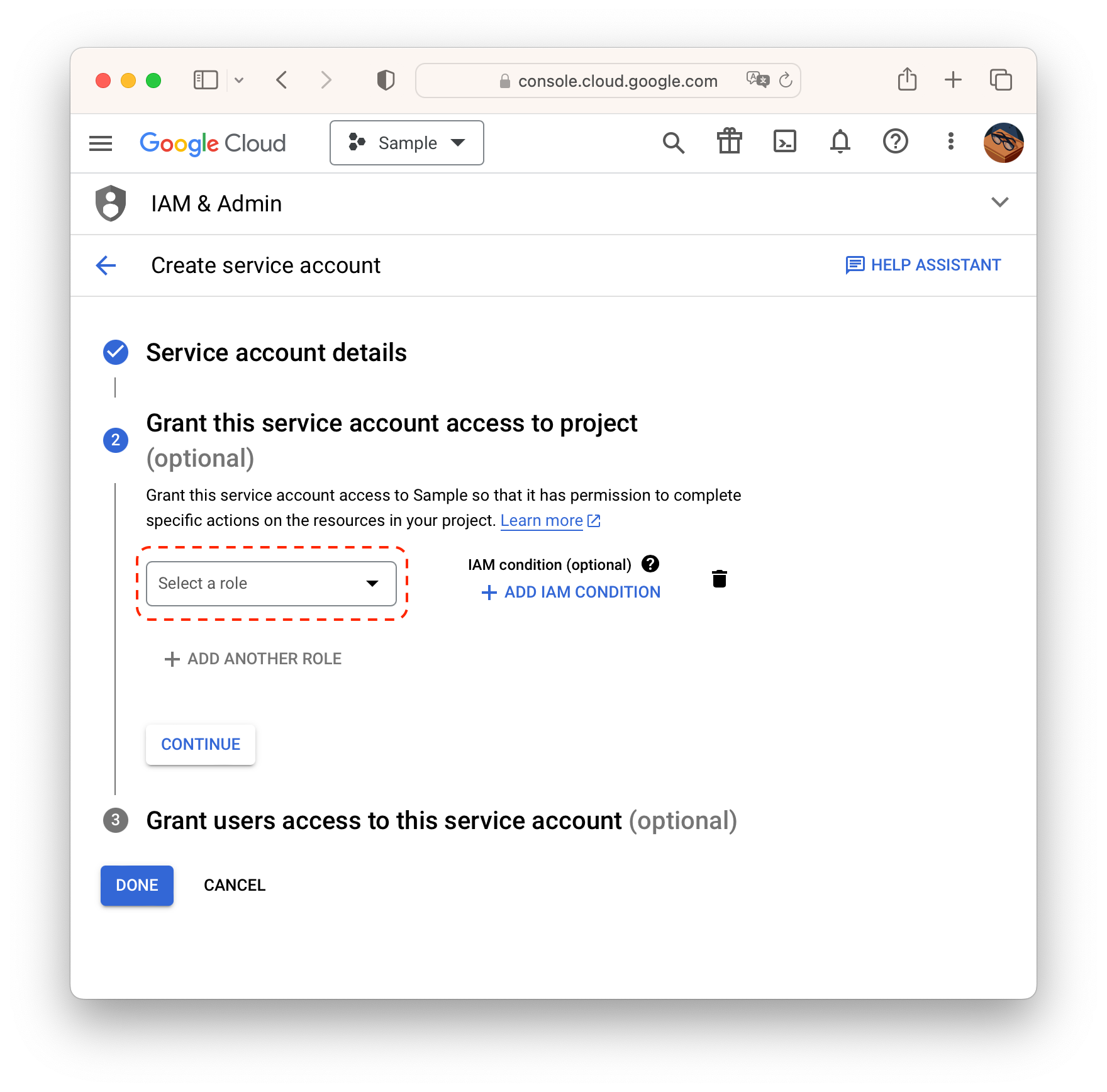
- Select a role created at step 6.
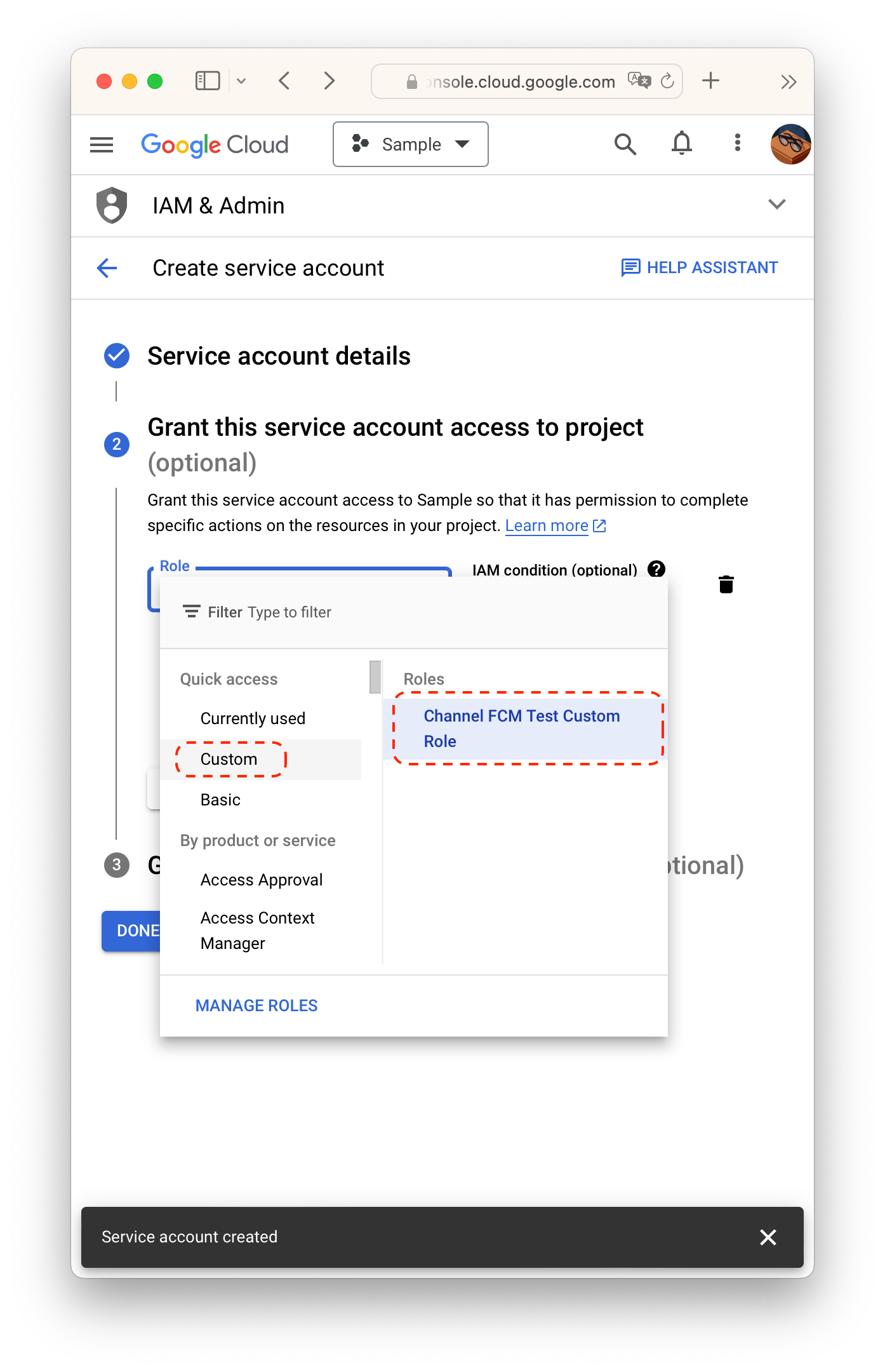
- Navigate to Manage keys for the service account.
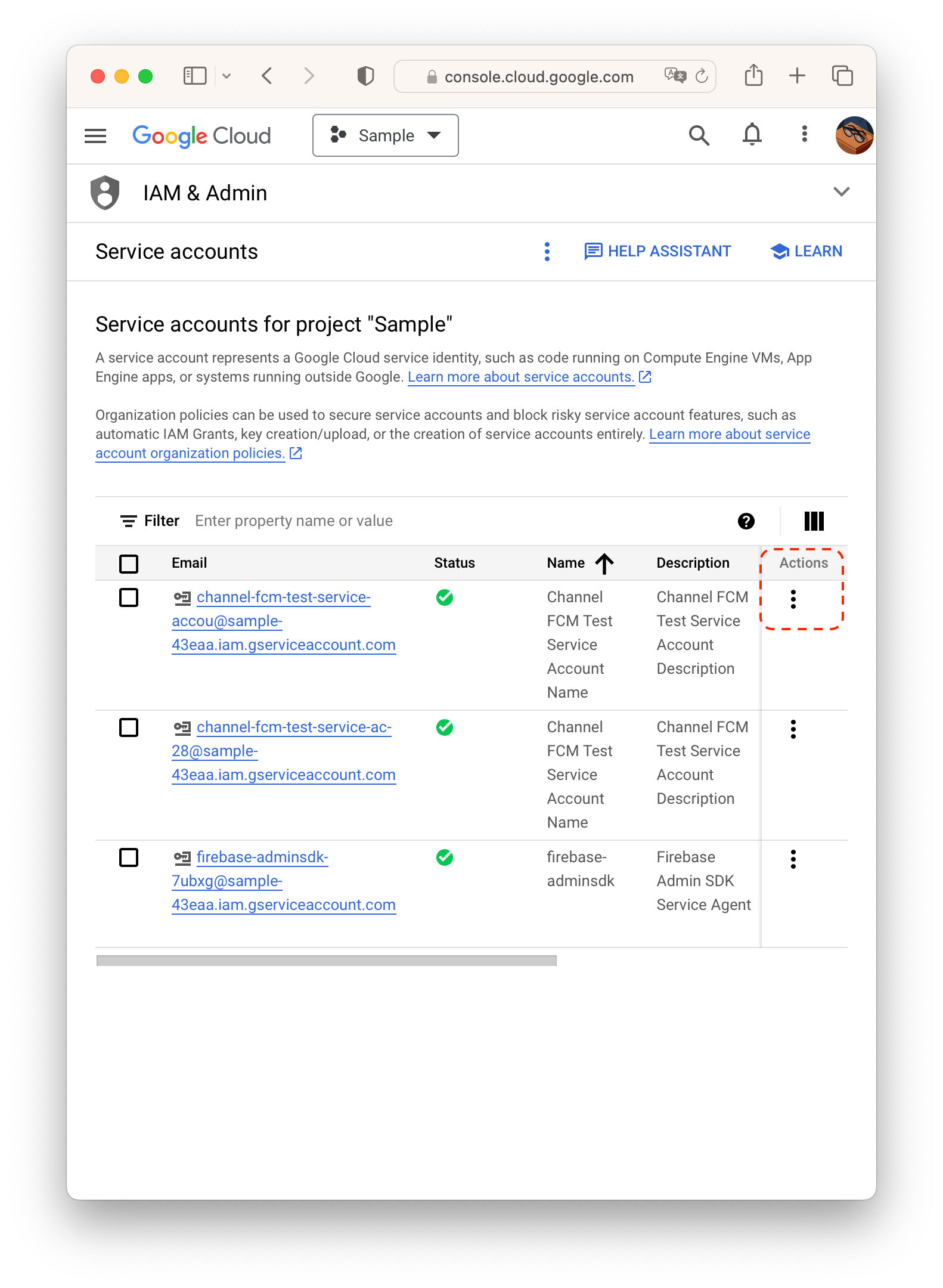
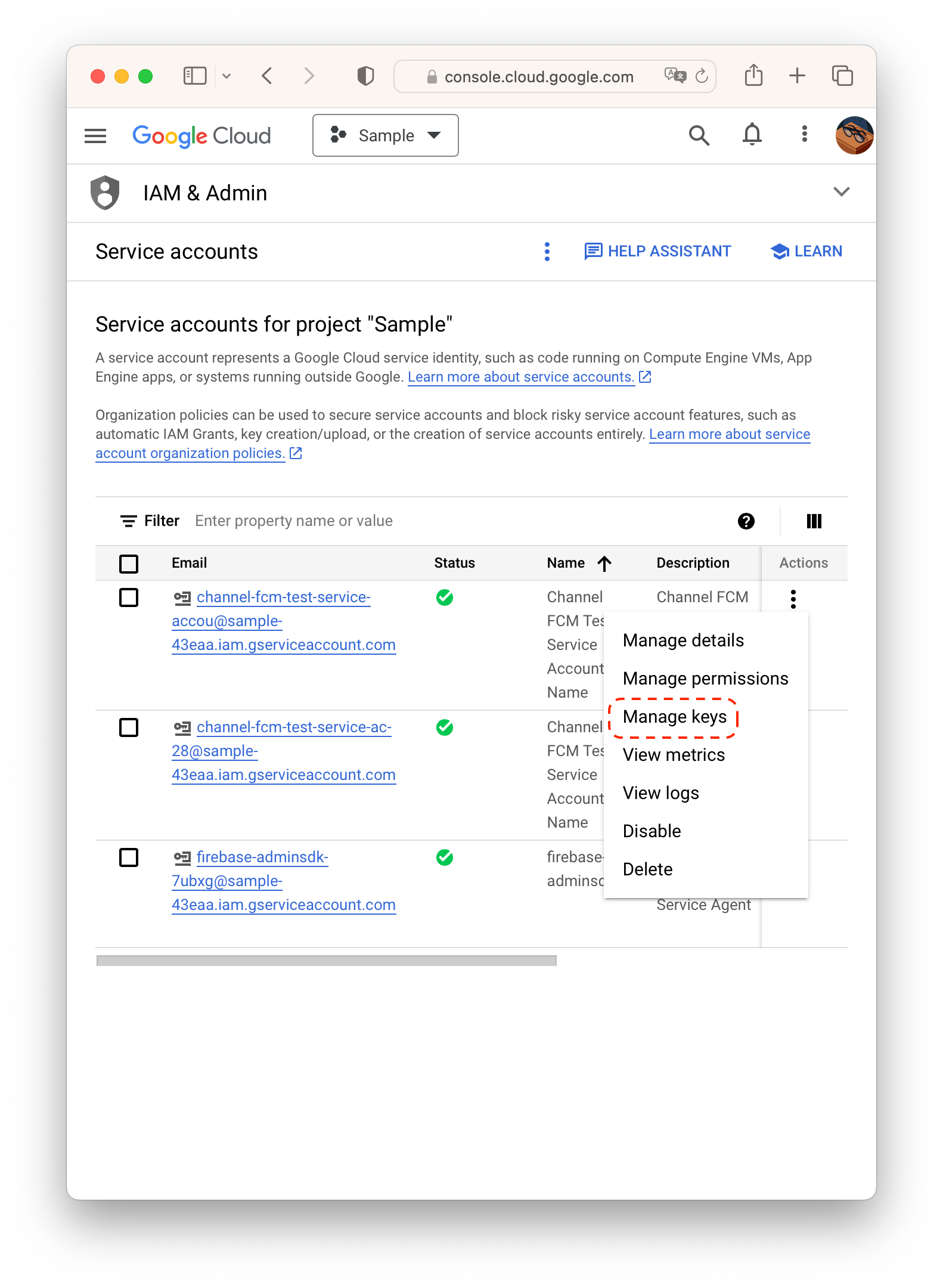
- Create a key by ADD KEY > Create new key with JSON format.
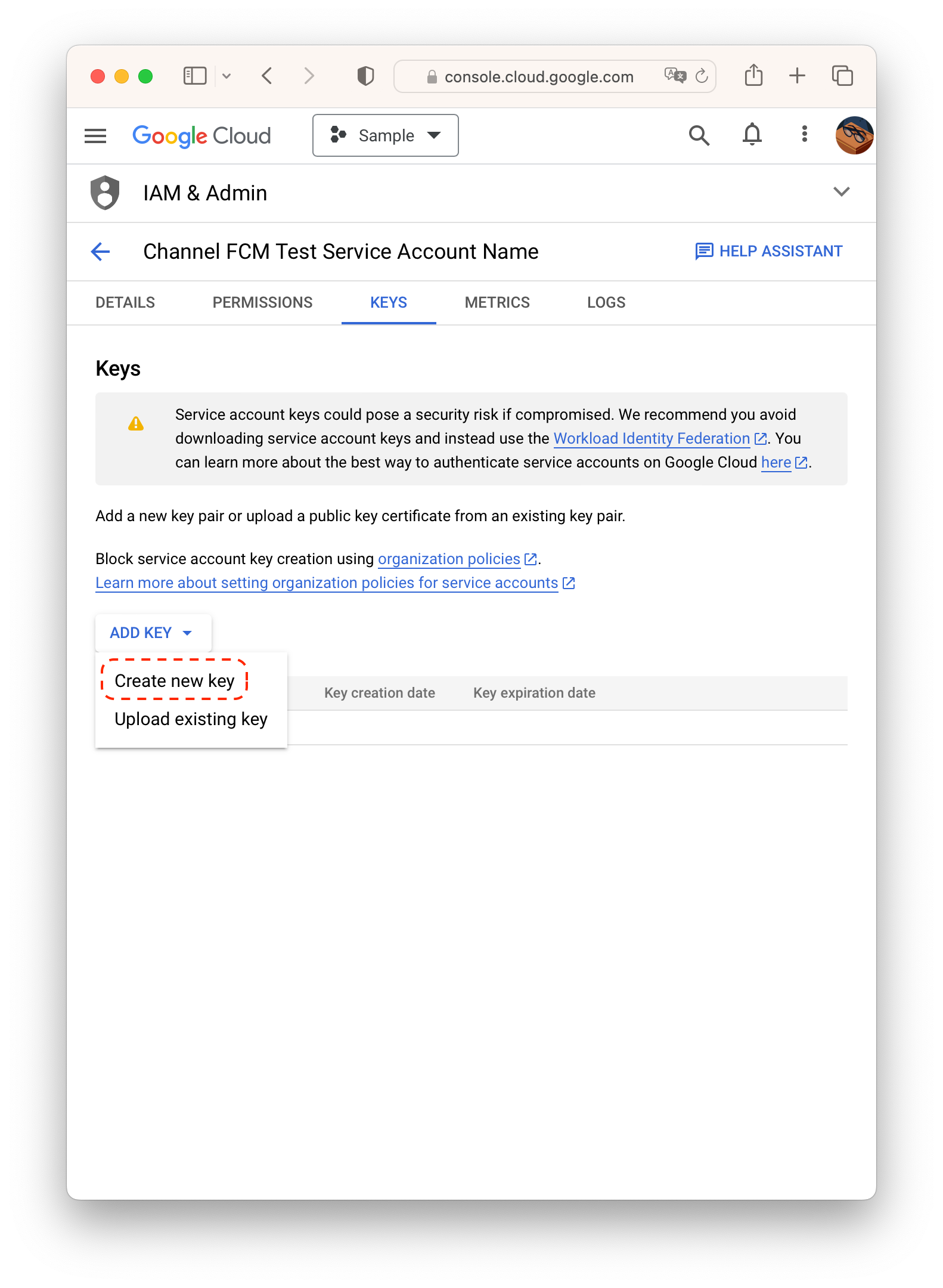
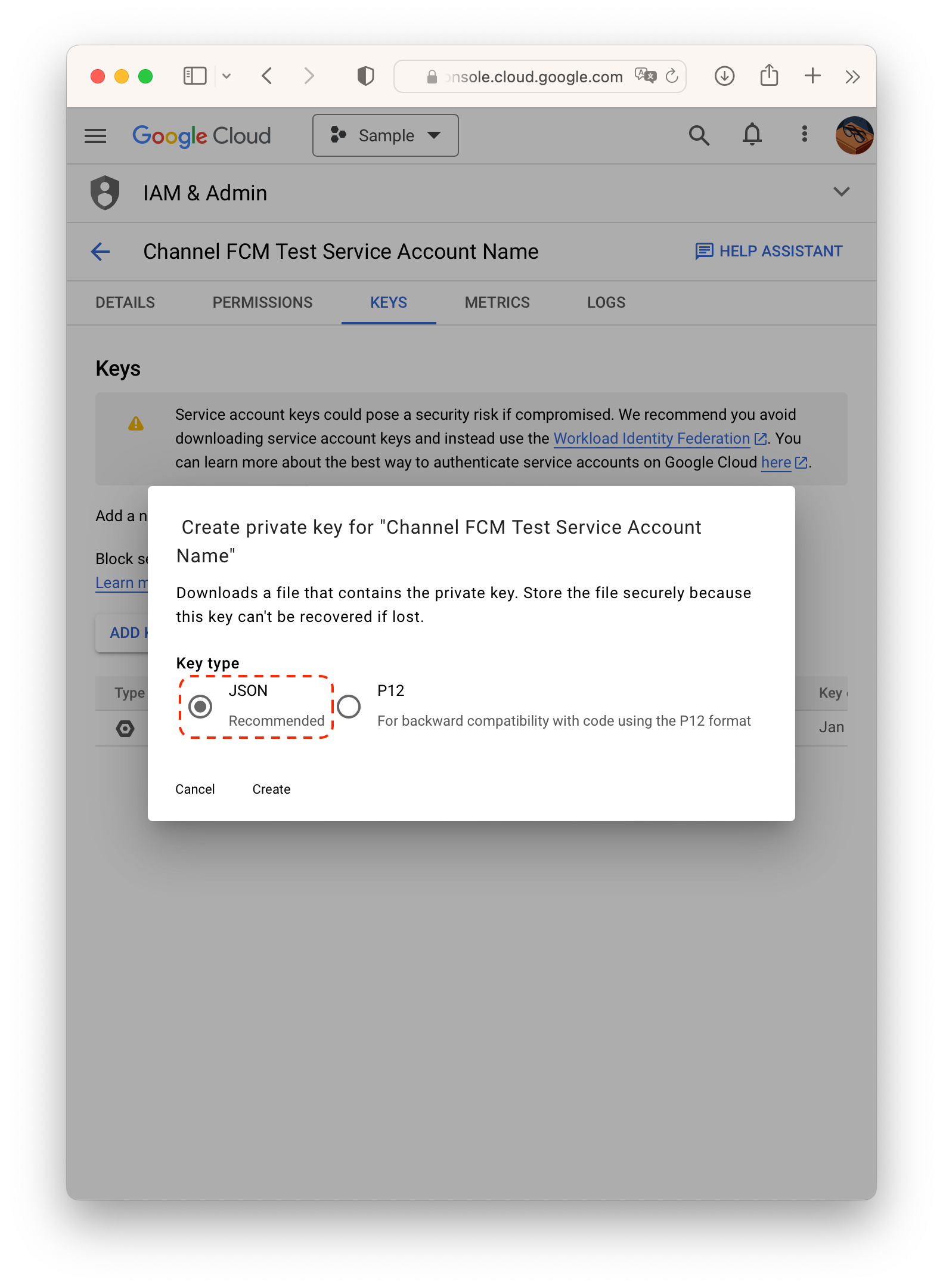
- Upload the key file to Channel Desk at Channel Settings > Security and Development > Mobile SDK Push > Android
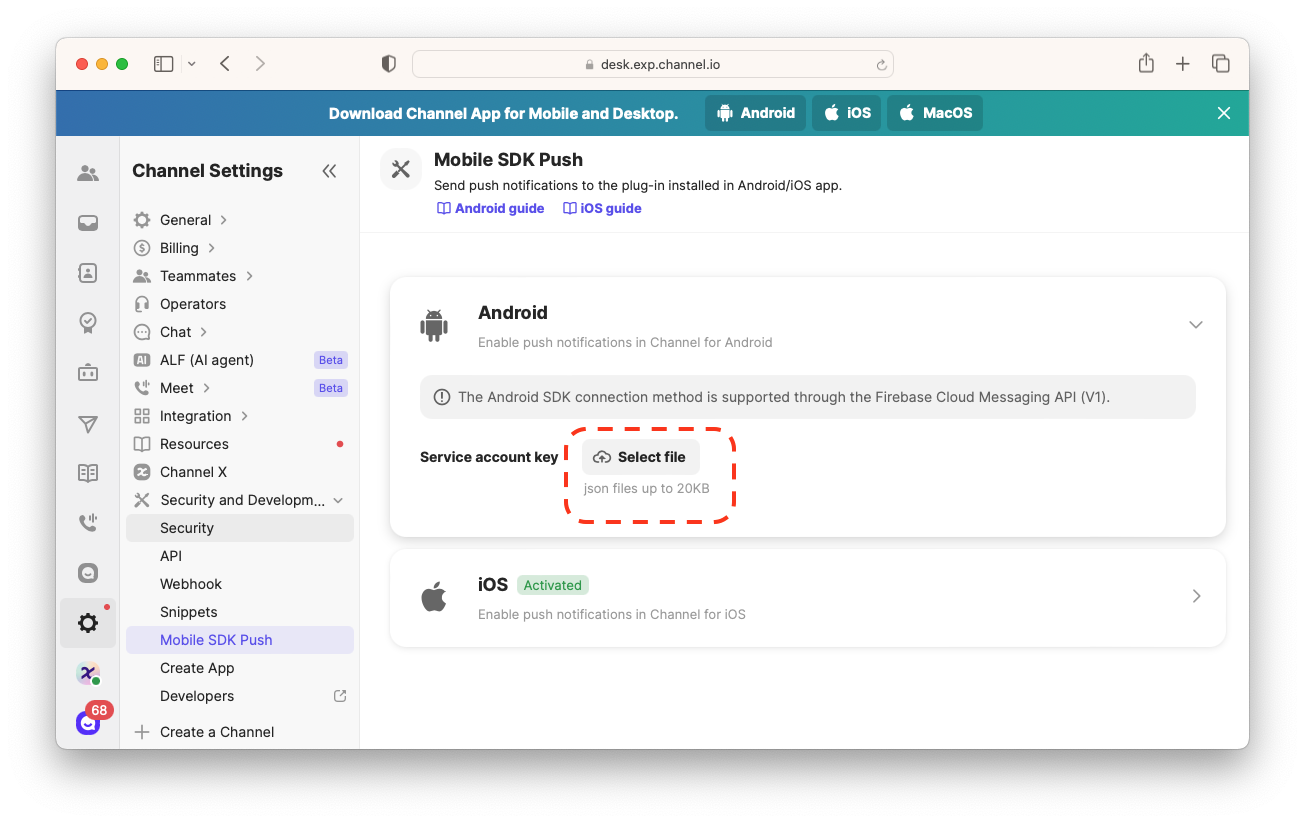
Customizing push notification
Changing a push title
You can set the title of a push notification by changing the value of notification_title
in strings.xml
. The default value is the appโs name.
<resources>
<string name="notification_title">Your notification title</string>
</resources>
Changing a push icon
If you wish to change the push icon, place the image file on the following path:
/res/drawable/ch_push_icon.png
Updated 8 months ago