1. Function이란 무엇인가
채널톡에서 앱의 기능을 요청하기 위해 사용하는 프로토콜입니다. http 위에서 json body 를 가지고 호출되며, body 의 method
, params
를 이용해 호출할 rpc 의 이름과 파라미터를 식별합니다. 앱으로 오고 가는 모든 요청은 Function
스키마를 따라 전송됩니다.
2. Function Handling
Function 이 호출될 경우 개발자 페이지에 등록하신Function Endpoint
로 HTTP PUT 요청을 전송하게 됩니다.
Function Endpoint
는 [앱 설정]
- [기본 설정]
- [서버 설정]
에 등록할 수 있습니다.
요청을 받은 경우 Signing Key
를 발급받아 요청이 정당한 소스 (채널톡) 으로부터 온 것인지 검증할 수 있습니다.
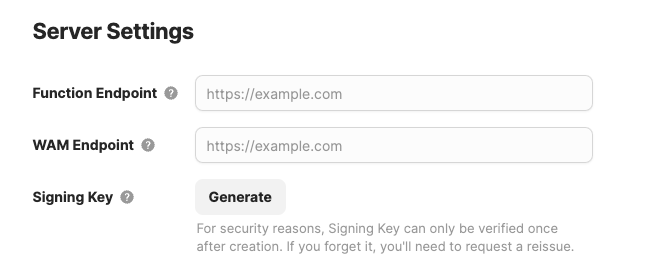
앱 서버에서는 이러한 요청을 처리하고 응답을 할 수 있는 HTTP API 를 Function Endpoint
위에서 제공해야 합니다.
method | url | notes |
---|---|---|
PUT | {Function Endpoint} | 채널톡 → 앱 서버로 호출되는 요청입니다 |
2.1. Function Request
Body
{
"method": "METHOD_NAME",
"params": {
"YOUR_ARGS[0]": "PROVIDED_PARAMS[0]",
"YOUR_ARGS[1]": "PROVIDED_PARAMS[1]",
...
},
"context": {
"channel": { "id": "CHANNEL_ID" },
"caller": { "type": "CALLER_TYPE", "id": "CALLER_ID" }
}
}
name | type | notes |
---|---|---|
method | string | function 으로 호출할 특정 기능의 이름 (rpc name) |
params | array or object | function body. function 에 전송할 json 데이터 |
context | object | function이 호출된 컨택스트 정보. 아래에 기술 |
context 는 function
의 호출자 정보를 담고 있습니다.
후술한 x-Signature
검증을 수행하는 경우 context
에 담긴 정보는 신뢰 가능합니다
field | type | notes | |
---|---|---|---|
channel | object | 호출된 채널 아이디 | |
caller | object | type : 호출자의 타입 - app , user , manager id : 호출자의 아이디 |
Header
notes | name | required |
---|---|---|
Signing Key 를 이용한 HMAC 서명 | X-Signature | true |
- signing key를 hexadecimal bytes로 encode 합니다.
- request body는 UTF-8 bytes로 encode 합니다.
- 1번에서 구한 시크릿 키와 HMAC SHA-256 함수로 2번에서 구한 값의 다이제스트를 구합니다.
- 다이제스트를 base64로 encode 합니다.
- header의 X-Signature 값과 4번에서 구한 값이 같은지 비교합니다.
// Java 예시
String signingKey = "..."; // signing key
String requestBody = "..."; // request body string
SecretKeySpec key = new SecretKeySpec(DatatypeConverter.parseHexBinary(signingKey), "HmacSHA256");
Mac mac = Mac.getInstance("HmacSHA256");
mac.init(key);
byte[] source = requestBody.getBytes(StandardCharsets.UTF_8);
byte[] digest = mac.doFinal(source);
String signature = Base64.encodeBase64String(digest);
// x-Signature header와 signature 비교
2.2. Function Response
field | type | notes |
---|---|---|
result | object or array | 요청 처리 성공시 응답값을 가진 필드 |
error | object | 요청 에러시 에러 오브젝트 |
성공 여부에 따라 result
또는 error
를 반환해야 합니다.
Success
{
"result": {
...
}
}
Failure
{
"error": {
"type": "..."
"message": "..."
}
}
field | type | notes |
---|---|---|
type | string | 에러 타입 |
message | string | 에러 메세지 |
3. Function Calling
앱에서 다른 앱의 function
역시 호출할 수 있습니다.
호출할 앱의 ID 와 앞서 설명한 Function
형식을 이용해 채널톡 api 서버로 요청을 전송합니다.
method | url | notes |
---|---|---|
PUT | app-store-api.channel.io/general/v1/apps/{appID}/functions | 채널에 설치된 다른 앱의 함수를 호출합니다 |
3.1. Function Request
Header
name | notes | required |
---|---|---|
x-access-token | 인증 및 권한에서 발급받은 채널 토큰 | true |
Body
{
"method": "METHOD_NAME",
"params": {
"YOUR_ARGS[0]": "PROVIDED_PARAMS[0]",
"YOUR_ARGS[1]": "PROVIDED_PARAMS[1]",
...
}
}
field | type | notes |
---|---|---|
method | string | function 으로 호출할 기능의 이름 (rpc name) |
params | array or object | function body. function 에 전송할 json 데이터 |
3.2. Function Response
성공 여부에 따라 result
혹은 error
가 반환됩니다
field | type | notes |
---|---|---|
result | array or object | 요청 처리 성공시 응답값을 가진 필드 |
error | object | 요청 에러시 에러 오브젝트 |
Success
{
"result": {
...
}
}
Failure
{
"error": {
"type": "...",
"message": "..."
}
}
field | type | notes |
---|---|---|
type | string | 에러 타입 |
message | string | 에러 메세지 |
4. Native Function Calling
Native Function
이란 채널톡에서 기본적으로 제공되는 Function
목록입니다. 유저챗에 메세지를 쓰거나, 매니저의 정보를 조회하는 등 채널톡 리소스를 조작하기 위해 사용합니다. 앱에서 Native Function
을 호출 하는 방법은 아래와 같습니다.
url | app-store-api.channel.io/general/v1/native/functions |
---|---|
method | PUT |
notes | 채널톡 Native Function 을 호출합니다 |
4.1. Native Function Request
Header
name | notes | required |
---|---|---|
x-access-token | 인증 및 권한 에서 발급받은 채널 토큰 | true |
Body
{
"method": "METHOD_NAME",
"params": {
"YOUR_ARGS[0]": "PROVIDED_PARAMS[0]",
"YOUR_ARGS[1]": "PROVIDED_PARAMS[1]",
...
}
}
field | type | notes |
---|---|---|
method | string | function 으로 호출할 특정 기능의 이름 (rpc name) |
params | array or object | function body. function 에 전송할 json 데이터 |
4.2. Native Function Response
성공 여부에 따라 result
또는 error
가 반환됩니다
field | type | notes |
---|---|---|
result | object | 요청 처리 성공시 응답값을 가진 필드 |
error | object | 요청 에러시 에러 오브젝트 |
Success
{
"result": {
...
}
}
Failure
{
"error": {
"type": "..."
"message": "..."
}
}
field | type | notes |
---|---|---|
type | string | 에러 타입 |
message | string | 에러 메세지 |
5. 현재 제공되는 Native Functions
App
registerCommands
request body
{
"method": "registerCommands",
"params": {
"appId": "...",
"commands": [
"name": "...",
"scope": "...",
"description": "...",
"nameDescI18nMap": {
"en": {
"name": "...",
"description": "..."
},
},
"actionFunctionName": "...",
"autoCompleteFunctionName": "..."
"paramDefinitions": [
{
"name": "...",
"type": "...", // "string", "float", "int", "bool"
"required": false,
"description": "...", // nullable
"choices": [ // nullable
{
"name": "...",
"value": { // any
},
"nameDescI18nMap": { // nullable
"en": {
},
}
},
],
"nameDescI18nMap": { // nullable
"en": {
},
}
"autoComplete": false, // nullable
"alfDescription": "..." // nullable
},
],
"enabledByDefault": true
]
}
}
response body
{}
Channel
writeGroupMessage
request body
{
"method": "writeGroupMessage",
"params": {
"channelId": "...",
"groupId": "...",
"rootMessageId": "...",
"broadcast": false,
"dto": {
"blocks": [
{ },
],
"plainText": "...",
"buttons": [
{
"title": "...",
"colorVariant": "...",
"action": { // one of commandAction, webAction, wamAction
"commandAction": { // option 1
"attributes" : {
"appId": "...",
"name": "...",
"params": {
}
}
}
"webAction": { // option 2
"attributes" : {
"url": "..."
}
}
"wamAction": { // option 3
"attributes" : {
"appId": "...",
"clientId": "...",
"name": "...",
"wamArgs": {
}
}
}
}
},
],
"files": [
{
"url": "...",
"mime": "...",
"fileName": "..."
},
],
"options": [
"...",
],
"requestId": "...",
"botName": "..."
}
}
}
response body
{
"result": {
"message": {
"id": "...",
"channelId": "...",
"chatType": "...",
"chatId": "...",
"personType": "...",
"personId": "...",
"requestId": "...",
"language": "...",
"createdAt": ,
"version": ,
"blocks": [
{ },
],
"plainText": "...",
"updatedAt": ,
"inboundEmailId": "...",
"thread": {
"id": "...",
"managerIds": [
"...",
],
"repliedManagerIds": [
"...",
],
"replyCount":
},
"buttons": [
{
"title": "...",
"colorVariant": "...",
"action": { // one of commandAction, webAction, wamAction
"commandAction": { // option 1
"attributes" : {
"appId": "...",
"name": "...",
"params": {
}
}
}
"webAction": { // option 2
"attributes" : {
"url": "..."
}
}
"wamAction": { // option 3
"attributes" : {
"appId": "...",
"clientId": "...",
"name": "...",
"wamArgs": {
}
}
}
}
},
],
"files": [
{
"url": "...",
"mime": "...",
"fileName": "..."
},
],
"state": "...",
"options": [
"...",
]
}
}
}
writeUserChatMessage
request body
{
"method": "writeUserChatMessage",
"params": {
"channelId": "...",
"userChatId": "...",
"dto": {
"blocks": [
{ },
],
"plainText": "...",
"buttons": [
{
"title": "...",
"colorVariant": "...",
"action": { // one of commandAction, webAction, wamAction
"commandAction": { // option 1
"attributes" : {
"appId": "...",
"name": "...",
"params": {
}
}
}
"webAction": { // option 2
"attributes" : {
"url": "..."
}
}
"wamAction": { // option 3
"attributes" : {
"appId": "...",
"clientId": "...",
"name": "...",
"wamArgs": {
}
}
}
}
},
],
"files": [
{
"url": "...",
"mime": "...",
"fileName": "..."
},
],
"options": [
"...",
],
"requestId": "...",
"botName": "..."
}
}
}
response body
{
"result": {
"message": {
"id": "...",
"channelId": "...",
"chatType": "...",
"chatId": "...",
"personType": "...",
"personId": "...",
"requestId": "...",
"language": "...",
"createdAt": ,
"version": ,
"blocks": [
{ },
],
"plainText": "...",
"updatedAt": ,
"inboundEmailId": "...",
"thread": {
"id": "...",
"managerIds": [
"...",
],
"repliedManagerIds": [
"...",
],
"replyCount":
},
"buttons": [
{
"title": "...",
"colorVariant": "...",
"action": { // one of commandAction, webAction, wamAction
"commandAction": { // option 1
"attributes" : {
"appId": "...",
"name": "...",
"params": {
}
}
}
"webAction": { // option 2
"attributes" : {
"url": "..."
}
}
"wamAction": { // option 3
"attributes" : {
"appId": "...",
"clientId": "...",
"name": "...",
"wamArgs": {
}
}
}
}
},
],
"files": [
{
"url": "...",
"mime": "...",
"fileName": "..."
},
],
"state": "...",
"options": [
"...",
]
}
}
}
getManager
request body
{
"method": "getManager",
"params": {
"channelId": "...",
"managerId": "..."
}
}
response body
{
"result": {
"manager": {
"id": "...",
"channelId": "...",
"accountId": "...",
"name": "...",
"description": "...",
"showDescriptionToFront": false,
"nameDescI18nMap": {
"en": {
"name": "...",
"description": "..."
},
},
"profile": {
},
"email": "...",
"showEmailToFront": false,
"roleId": "...",
"removed": false,
"createdAt": ,
"UpdatedAt": ,
"displayAsChannel": false,
"defaultGroupWatch": "...",
"defaultDirectChatWatch": "...",
"defaultUserChatWatch": "...",
"chatAlertSound": "...",
"meetAlertSound": "...",
"showPrivateMessagePreview": false,
"operatorScore": ,
"touchScore": ,
"operatorEmailReminder": false,
"receiveUnassignedChatAlert": false,
"receiveUnassignedMeetAlert": false,
"operator": false,
"defaultAllMentionImportant": false,
"userMessageImportant": false,
"autoAssignCapacity": ,
"statusEmoji": "...",
"statusText": "...",
"statusClearAt": ,
"doNotDisturb": false,
"doNotDisturbClearAt": ,
"accountDoNotDisturb": false,
"accountDoNotDisturbClearAt": ,
"enableReactedMessageIndex": false,
"operatorUpdatedAt": ,
"avatarUrl": "...",
"emailForFront": "...",
"mobileNumberForFront": "...",
"meetOperator": false
}
}
}
batchGetManagers
request body
{
"method": "batchGetManagers",
"params": {
"channelId": "...",
"managerIds": [ number of ids min: 1 max: 50
"...",
]
}
}
response body
{
"result": {
"managers": [
{
"id": "...",
"channelId": "...",
"accountId": "...",
"name": "...",
"description": "...",
"showDescriptionToFront": false,
"nameDescI18nMap": {
"en": {
"name": "...",
"description": "..."
},
},
"profile": {
},
"email": "...",
"showEmailToFront": false,
"roleId": "...",
"removed": false,
"createdAt": ,
"UpdatedAt": ,
"displayAsChannel": false,
"defaultGroupWatch": "...",
"defaultDirectChatWatch": "...",
"defaultUserChatWatch": "...",
"chatAlertSound": "...",
"meetAlertSound": "...",
"showPrivateMessagePreview": false,
"operatorScore": ,
"touchScore": ,
"operatorEmailReminder": false,
"receiveUnassignedChatAlert": false,
"receiveUnassignedMeetAlert": false,
"operator": false,
"defaultAllMentionImportant": false,
"userMessageImportant": false,
"autoAssignCapacity": ,
"statusEmoji": "...",
"statusText": "...",
"statusClearAt": ,
"doNotDisturb": false,
"doNotDisturbClearAt": ,
"accountDoNotDisturb": false,
"accountDoNotDisturbClearAt": ,
"enableReactedMessageIndex": false,
"operatorUpdatedAt": ,
"avatarUrl": "...",
"emailForFront": "...",
"mobileNumberForFront": "...",
"meetOperator": false
},
]
}
}
searchManagers
request body
{
"method": "searchManagers",
"params": {
"channelId": "...",
"pagination": {
"sortOrder": "...",
"since": "...",
"limit":
}
}
}
response body
{
"result": {
"managers": [
{
"id": "...",
"channelId": "...",
"accountId": "...",
"name": "...",
"description": "...",
"showDescriptionToFront": false,
"nameDescI18nMap": {
"en": {
"name": "...",
"description": "..."
},
},
"profile": {
},
"email": "...",
"showEmailToFront": false,
"roleId": "...",
"removed": false,
"createdAt": ,
"UpdatedAt": ,
"displayAsChannel": false,
"defaultGroupWatch": "...",
"defaultDirectChatWatch": "...",
"defaultUserChatWatch": "...",
"chatAlertSound": "...",
"meetAlertSound": "...",
"showPrivateMessagePreview": false,
"operatorScore": ,
"touchScore": ,
"operatorEmailReminder": false,
"receiveUnassignedChatAlert": false,
"receiveUnassignedMeetAlert": false,
"operator": false,
"defaultAllMentionImportant": false,
"userMessageImportant": false,
"autoAssignCapacity": ,
"statusEmoji": "...",
"statusText": "...",
"statusClearAt": ,
"doNotDisturb": false,
"doNotDisturbClearAt": ,
"accountDoNotDisturb": false,
"accountDoNotDisturbClearAt": ,
"enableReactedMessageIndex": false,
"operatorUpdatedAt": ,
"avatarUrl": "...",
"emailForFront": "...",
"mobileNumberForFront": "...",
"meetOperator": false
},
],
"next": "..."
}
}
getUserChat
request body
{
"method": "getUserChat",
"params": {
"channelId": "...",
"userChatId": "..."
}
}
response body
{
"result": {
"userChat": {
"id" : "...",
"channelId" : "...",
"contactMediumType" : "...",
"liveMeetId" : "...",
"sync" : false,
"state" : "...",
"missedReason" : "...",
"managed" : false,
"userId" : "...",
"xerId" : "...",
"name" : "...",
"title" : "...",
"description" : "...",
"subtextType" : "...",
"handling" : {
},
"source" : {
},
"managerIds" : [
"...",
],
"assigneeId" : "...",
"teamId" : "...",
"tags" : [
"...",
],
"goalEventName" : "...",
"goalEventQuery" : {
},
"goalCheckedAt" : ,
"goalState" : "...",
"firstOpenedAt" : ,
"openedAt" : ,
"createdAt" : ,
"updatedAt" : ,
"frontMessageId" : "...",
"frontUpdatedAt" : ,
"deskMessageId" : "...",
"deskUpdatedAt" : ,
"firstAssigneeIdAfterOpen" : "...",
"firstRepliedAtAfterOpen" : ,
"oneStop" : false,
"waitingTime" : ,
"avgReplyTime" : ,
"totalReplyTime" : ,
"replyCount" : ,
"resolutionTime" : ,
"operationWaitingTime" : ,
"operationAvgReplyTime" : ,
"operationTotalReplyTime" : ,
"operationReplyCount" : ,
"operationResolutionTime" : ,
"firstAskedAt" : ,
"askedAt" : ,
"closedAt" : ,
"snoozedAt" : ,
"expiresAt" : ,
"version" :
}
}
}
getUser
request body
{
"method": "getUser",
"params": {
"channelId": "...",
"userId": "..."
}
}
response body
{
"result": {
"user": {
"id" : "...",
"channelId" : "...",
"memberId" : "...",
"veilId" : "...",
"unifiedId" : "...",
"type" : "...",
"name" : "...",
"mobileNumberQualified" : false,
"emailQualified" : false,
"profile" : {
},
"tags" : [
"...",
],
"alert" : ,
"unread" : ,
"popUpChatId" : "...",
"blocked" : false,
"unsubscribeEmail" : false,
"unsubscribeTexting" : false,
"hasChat" : false,
"mainChatId" : "...",
"hasPushToken" : false,
"language" : "...",
"country" : "...",
"timeZone" : "...",
"province" : "...",
"city" : "...",
"latitude" : ,
"longitude" : ,
"web" : {
},
"mobile" : {
},
"sessionsCount" : ,
"lastSeenAt" : ,
"createdAt" : ,
"updatedAt" : ,
"version" :
}
}
}
getChannel
request body
{
"method": "getChannel",
"params": {
"channelId": "..."
}
}
response body
{
"result": {
"channel": {
"id" : "...",
"createdAt" : ,
"updatedAt" : ,
"state" : "...",
"blocked" : false,
"name" : "...",
"description" : "...",
"nameDescI18nMap" : {
"en": {
"name": "...",
"description": "..."
},
},
"botName" : "...",
"color" : "...",
"country" : "...",
"domain" : "...",
"systemDomain" : "...",
"homepageUrl" : "...",
"phoneNumber" : "...",
"timezone" : "...",
"utcOffset" : "...",
"bizCertificated" : false,
"bizCertificatedCountries" : [
"...",
],
"defaultPluginId" : "...",
"welcomeMessage" : {
},
"welcomeMessageI18nMap" : {
"en": {
},
},
"expectedResponseDelay" : "...",
"showOperatorProfile" : false,
"inOperation" : false,
"working" : false,
"operationTimeScheduling" : false,
"nextWorkingTime" : ,
"nextAwayTime" : ,
"nextOperatingAt" : ,
"operationTimeRanges" : [
{
"dayOfWeeks": [
"...",
],
"from": ,
"to":
},
],
"awayOption" : "...",
"blockReplyingAfterClosed" : false,
"blockReplyingAfterClosedTime" : ,
"followUpTexting" : false,
"followUpEmail" : false,
"followUpAskName" : false,
"followUpMandatory" : false,
"avatarUrl" : "...",
"initial" : "...",
"borderColor" : "...",
"gradientColor" : "...",
"textColor" : "...",
"brightness" : ,
"coverImageColor" : "...",
"coverImageUrl" : "...",
"coverImageBright" : false,
"appCommerceId" : "...",
"appCommerceType" : "...",
"appCommerceDomain" : "...",
"defaultEmailDomainId" : "...",
"hideAppMessenger" : false,
"userInfoUrl" : "...",
"enableMemberHash" : false,
"enableMfa" : false,
"indebtedDueDate" : "...",
"entVerified" : false,
"sourceSurvey" : {
},
"bizCategory" : "...",
"staffs" : ,
"trafficSource" : {
},
"baseTutorialCompleted" : false,
"managedUserChatRetentionDuration" :
}
}
}
manageUserChat
request body
{
"method": "manageUserChat",
"params": {
"channelId": "...",
"userChatId": "..."
}
}
response body
{
"result": {
"userChat": {
"id" : "...",
"channelId" : "...",
"contactMediumType" : "...",
"liveMeetId" : "...",
"sync" : false,
"state" : "...",
"missedReason" : "...",
"managed" : false,
"userId" : "...",
"xerId" : "...",
"name" : "...",
"title" : "...",
"description" : "...",
"subtextType" : "...",
"handling" : {
},
"source" : {
},
"managerIds" : [
"...",
],
"assigneeId" : "...",
"teamId" : "...",
"tags" : [
"...",
],
"goalEventName" : "...",
"goalEventQuery" : {
},
"goalCheckedAt" : ,
"goalState" : "...",
"firstOpenedAt" : ,
"openedAt" : ,
"createdAt" : ,
"updatedAt" : ,
"frontMessageId" : "...",
"frontUpdatedAt" : ,
"deskMessageId" : "...",
"deskUpdatedAt" : ,
"firstAssigneeIdAfterOpen" : "...",
"firstRepliedAtAfterOpen" : ,
"oneStop" : false,
"waitingTime" : ,
"avgReplyTime" : ,
"totalReplyTime" : ,
"replyCount" : ,
"resolutionTime" : ,
"operationWaitingTime" : ,
"operationAvgReplyTime" : ,
"operationTotalReplyTime" : ,
"operationReplyCount" : ,
"operationResolutionTime" : ,
"firstAskedAt" : ,
"askedAt" : ,
"closedAt" : ,
"snoozedAt" : ,
"expiresAt" : ,
"version" :
}
}
}
Manager
writeGroupMessageAsManager
request body
{
"method": "writeGroupMessageAsManager",
"params": {
"channelId": "...",
"groupId": "...",
"rootMessageId": "...",
"broadcast": false,
"dto": {
"blocks": [
{ },
],
"plainText": "...",
"buttons": [
{
"title": "...",
"colorVariant": "...",
"action": { // one of commandAction, webAction, wamAction
"commandAction": { // option 1
"attributes" : {
"appId": "...",
"name": "...",
"params": {
}
}
}
"webAction": { // option 2
"attributes" : {
"url": "..."
}
}
"wamAction": { // option 3
"attributes" : {
"appId": "...",
"clientId": "...",
"name": "...",
"wamArgs": {
}
}
}
}
},
],
"files": [
{
"url": "...",
"mime": "...",
"fileName": "..."
},
],
"options": [
"...",
],
"requestId": "...",
"managerId": "..."
}
}
}
response body
{
"result": {
"message": {
"id": "...",
"channelId": "...",
"chatType": "...",
"chatId": "...",
"personType": "...",
"personId": "...",
"requestId": "...",
"language": "...",
"createdAt": ,
"version": ,
"blocks": [
{ },
],
"plainText": "...",
"updatedAt": ,
"inboundEmailId": "...",
"thread": {
"id": "...",
"managerIds": [
"...",
],
"repliedManagerIds": [
"...",
],
"replyCount":
},
"buttons": [
{
"title": "...",
"colorVariant": "...",
"action": { // one of commandAction, webAction, wamAction
"commandAction": { // option 1
"attributes" : {
"appId": "...",
"name": "...",
"params": {
}
}
}
"webAction": { // option 2
"attributes" : {
"url": "..."
}
}
"wamAction": { // option 3
"attributes" : {
"appId": "...",
"clientId": "...",
"name": "...",
"wamArgs": {
}
}
}
}
},
],
"files": [
{
"url": "...",
"mime": "...",
"fileName": "..."
},
],
"state": "...",
"options": [
"...",
]
}
}
}
writeUserChatMessageAsManager
request body
{
"method": "writeUserChatMessageAsManager",
"params": {
"channelId": "...",
"userChatId": "...",
"dto": {
"blocks": [
{ },
],
"plainText": "...",
"buttons": [
{
"title": "...",
"colorVariant": "...",
"action": { // one of commandAction, webAction, wamAction
"commandAction": { // option 1
"attributes" : {
"appId": "...",
"name": "...",
"params": {
}
}
}
"webAction": { // option 2
"attributes" : {
"url": "..."
}
}
"wamAction": { // option 3
"attributes" : {
"appId": "...",
"clientId": "...",
"name": "...",
"wamArgs": {
}
}
}
}
},
],
"files": [
{
"url": "...",
"mime": "...",
"fileName": "..."
},
],
"options": [
"...",
],
"requestId": "...",
"managerId": "..."
}
}
}
response body
{
"result": {
"message": {
"id": "...",
"channelId": "...",
"chatType": "...",
"chatId": "...",
"personType": "...",
"personId": "...",
"requestId": "...",
"language": "...",
"createdAt": ,
"version": ,
"blocks": [
{ },
],
"plainText": "...",
"updatedAt": ,
"inboundEmailId": "...",
"thread": {
"id": "...",
"managerIds": [
"...",
],
"repliedManagerIds": [
"...",
],
"replyCount":
},
"buttons": [
{
"title": "...",
"colorVariant": "...",
"action": { // one of commandAction, webAction, wamAction
"commandAction": { // option 1
"attributes" : {
"appId": "...",
"name": "...",
"params": {
}
}
}
"webAction": { // option 2
"attributes" : {
"url": "..."
}
}
"wamAction": { // option 3
"attributes" : {
"appId": "...",
"clientId": "...",
"name": "...",
"wamArgs": {
}
}
}
}
},
],
"files": [
{
"url": "...",
"mime": "...",
"fileName": "..."
},
],
"state": "...",
"options": [
"...",
]
}
}
}
writeDirectChatMessageAsManager
request body
{
"method": "writeDirectChatMessageAsManager",
"params": {
"directChatId": "...",
"channelId": "...",
"rootMessageId": "...",
"broadcast": false,
"dto": {
"blocks": [
{ },
],
"plainText": "...",
"buttons": [
{
"title": "...",
"colorVariant": "...",
"action": { // one of commandAction, webAction, wamAction
"commandAction": { // option 1
"attributes" : {
"appId": "...",
"name": "...",
"params": {
}
}
}
"webAction": { // option 2
"attributes" : {
"url": "..."
}
}
"wamAction": { // option 3
"attributes" : {
"appId": "...",
"clientId": "...",
"name": "...",
"wamArgs": {
}
}
}
}
},
],
"files": [
{
"url": "...",
"mime": "...",
"fileName": "..."
},
],
"options": [
"...",
],
"requestId": "...",
"managerId": "..."
}
}
}
response body
{
"result": {
"message": {
"id": "...",
"channelId": "...",
"chatType": "...",
"chatId": "...",
"personType": "...",
"personId": "...",
"requestId": "...",
"language": "...",
"createdAt": ,
"version": ,
"blocks": [
{ },
],
"plainText": "...",
"updatedAt": ,
"inboundEmailId": "...",
"thread": {
"id": "...",
"managerIds": [
"...",
],
"repliedManagerIds": [
"...",
],
"replyCount":
},
"buttons": [
{
"title": "...",
"colorVariant": "...",
"action": { // one of commandAction, webAction, wamAction
"commandAction": { // option 1
"attributes" : {
"appId": "...",
"name": "...",
"params": {
}
}
}
"webAction": { // option 2
"attributes" : {
"url": "..."
}
}
"wamAction": { // option 3
"attributes" : {
"appId": "...",
"clientId": "...",
"name": "...",
"wamArgs": {
}
}
}
}
},
],
"files": [
{
"url": "...",
"mime": "...",
"fileName": "..."
},
],
"state": "...",
"options": [
"...",
]
}
}
}
User
writeUserChatMessageAsUser
request body
{
"method": "writeUserChatMessageAsUser",
"params": {
"channelId": "...",
"userChatId": "...",
"dto": {
"blocks": [
{ },
],
"plainText": "...",
"buttons": [
{
"title": "...",
"colorVariant": "...",
"action": { // one of commandAction, webAction, wamAction
"commandAction": { // option 1
"attributes" : {
"appId": "...",
"name": "...",
"params": {
}
}
}
"webAction": { // option 2
"attributes" : {
"url": "..."
}
}
"wamAction": { // option 3
"attributes" : {
"appId": "...",
"clientId": "...",
"name": "...",
"wamArgs": {
}
}
}
}
},
],
"files": [
{
"url": "...",
"mime": "...",
"fileName": "..."
},
],
"options": [
"...",
],
"requestId": "...",
"userId": "..."
}
}
}
response body
{
"result": {
"message": {
"id": "...",
"channelId": "...",
"chatType": "...",
"chatId": "...",
"personType": "...",
"personId": "...",
"requestId": "...",
"language": "...",
"createdAt": ,
"version": ,
"blocks": [
{ },
],
"plainText": "...",
"updatedAt": ,
"inboundEmailId": "...",
"thread": {
"id": "...",
"managerIds": [
"...",
],
"repliedManagerIds": [
"...",
],
"replyCount":
},
"buttons": [
{
"title": "...",
"colorVariant": "...",
"action": { // one of commandAction, webAction, wamAction
"commandAction": { // option 1
"attributes" : {
"appId": "...",
"name": "...",
"params": {
}
}
}
"webAction": { // option 2
"attributes" : {
"url": "..."
}
}
"wamAction": { // option 3
"attributes" : {
"appId": "...",
"clientId": "...",
"name": "...",
"wamArgs": {
}
}
}
}
},
],
"files": [
{
"url": "...",
"mime": "...",
"fileName": "..."
},
],
"state": "...",
"options": [
"...",
]
}
}
}